From the Web:
Michal Sramka's Matches Game
Michal Sramka has used JavaScript to produce one of the traditional
games used in many programming courses: the matches game. His
game is on-line at
http://www.sanet.sk/~ms/js/matches.html
The rules of the game are simple: There is a supply of matches-in
this case the number is selected by the user. The user and the
computer take turns taking one, two, or three matches in an attempt
to force the other player to take the last match. If you take
the last match, you lose. If the computer is forced to take it,
then you win.
In Sramka's example, he has developed a fairly sophisticated table-based
interface that makes extensive use of JavaScript.
Listing W5.1. Source code for Michal Sramka's Matches Game.
<html>
<head>
<title>JavaScript</title>
</head>
<body onload="welcome()" onunload="bye()">
<script language="JavaScript">
<!-- Begin
function welcome() {
document.game.help.value=" Please enter
number
of matches and click Start game.";
document.game.number.focus();
window.defaultStatus="JavaScript Safety Matches
Game"; }
function bye() {
window.defaultStatus=""; }
function come_on() {
if(document.game.number.value<5) alert("Must
be more than 4");
else {
document.game.help.value=" Wow,
it's your turn. Click 1, 2 or 3.";
document.game.count.value=document.game.number.value;
} }
function letsgo(yourchoice) {
var date=new Date(),mychoice;
if(document.game.count.value-yourchoice<=1) {
document.game.help.value=" You
win !";
document.game.count.value=1; }
else {
if(document.game.count.value%4==0) {
if(yourchoice==1) mychoice=2;
else {
if(date.getSeconds()%2==0)
mychoice=3;
else mychoice=1;
} }
else if(document.game.count.value%4==1)
mychoice=4-yourchoice;
else if(document.game.count.value%4==2)
{
if(yourchoice==2) mychoice=3;
else {
if(date.getSeconds()%2==0)
mychoice=1;
else mychoice=2;
} }
else {
if(yourchoice==1) mychoice=1;
else {
if(date.getSeconds()%2==0)
mychoice=2;
else mychoice=3;
} }
if(document.game.count.value-yourchoice<4)
mychoice=document.game.count.value-yourchoice-1;
document.game.count.value-=yourchoice+mychoice;
document.game.me.value=" "+mychoice;
if(document.game.count.value==1) document.game.help.value=
" I
took "+mychoice+" matches and win this game !";
else document.game.help.value=
" I
took "+mychoice+" matches. It's your turn again.";
} }
// End -->
</script>
<center>
<h1>Safety Matches Game</h1>
This is a very easy game. It's avaliable as JavaScript Applet
as well as
<a href="/~milan/JAVA/matches.html">Java Applet</a><br>
At first choose the number of matches. Then you have to take 1,
2 or 3
matches by clicking on the appropriate button. The Master will
do the
same. Who takes the last match - LOSE !<p>
<form name="game">
<table border="3" cellpadding="0" cellspacing="2">
<tr>
<td align="left">How many matches ?</td>
<td align="right"><input type="text"
name="number" size="10">
<input
type="button" name="start" value=" Start
game "
onclick="if(confirm('Really
start new game ?')) come_on()"></td>
</tr>
<tr>
<td align="center" colspan="2"><input
type="text" name="help" size="55"></td>
</tr>
<tr>
<td align="left">Counter:</td>
<td align="right"><input type="text"
name="count" size="10"></td>
</tr>
<tr>
<td align="left">Your Choice:</td>
<td align="right"><input type="button"
name="one"
value="
1 " onclick="letsgo(1)"><input type="button"
name="two"
value=" 2 " onclick="letsgo(2)">
<input
type="button" name="three" value=" 3
" onclick="letsgo(3)"></td>
</tr>
<tr>
<td align="left">My Choice:</td>
<td align="right"><input type="text"
name="me" size="3"></td>
</tr>
</table>
</form>
<hr><b><i><a href="/~ms/ms.html">Michal
Sramka</a>'s
<a
href="/~ms/js/">JavaScript Archive</a></i></b>
</center>
</body>
</html>
The script produces results similar to those in W5.1.
Figure w5.1: Michal Sramka's Matches Game.
 |
To understand Sramka's program, you need to start by looking at the table, which is the primary interface for the game.
|
<form name="game">
<table border="3" cellpadding="0" cellspacing="2">
<tr>
<td align="left">How many matches ?</td>
<td align="right"><input type="text"
name="number" size="10">
<input
type="button" name="start" value=" Start
game "
onclick="if(confirm('Really
start new game ?')) come_on()"></td>
</tr>
<tr>
<td align="center" colspan="2"><input
type="text" name="help" size="55"></td>
</tr>
<tr>
<td align="left">Counter:</td>
<td align="right"><input type="text"
name="count" size="10"></td>
</tr>
<tr>
<td align="left">Your Choice:</td>
<td align="right"><input type="button"
name="one" value=" 1 "
onclick="letsgo(1)"><input
type="button" name="two"
value="
2 " onclick="letsgo(2)"><input type="button"
name="three"
value=" 3 " onclick="letsgo(3)"></td>
</tr>
<tr>
<td align="left">My Choice:</td>
<td align="right"><input type="text"
name="me" size="3"></td>
</tr>
</table>
</form>
There is a Start button that confirms the user wants to start
and then calls come_on().
If a new game starts, it uses the number in the number
field to determine the number of matches in the game. The help
text field is the primary display point for messages about the
status of the game. The count
field is used to display the number of matches left in the current
game. There are three buttons that the user uses to select the
number of matches he wants to take each turn. The me
field is used to display the computer's choice each round.
There are four functions in the main script: welcome(),
bye(), come_on(),
and letsgo(). The welcome()
and bye() functions display
welcome and farewell messages and are invoked from the BODY
tag's onLoad and onUnload
event handler.
The come_on() function is
called when the user decides to start a new game.
function come_on() {
if(document.game.number.value<5) alert("Must
be more than 4");
else {
document.game.help.value=" Wow,
it's your turn. Click 1, 2 or 3.";
document.game.count.value=document.game.number.value;
} }
The function checks that the value in number
is valid (greater than or equal to 5).
If not, the user is alerted to choose another number. Otherwise,
the help field informs the
user that it is his turn, and the count
field is updated with the starting number of matches.
The real work of the game takes place in letsgo().
The function is invoked when the user clicks on one of the three
buttons to select a number of matches to take. The function takes
one argument: the number of matches selected by the user.
The first step is to declare the global variables and objects:
date and mychoice.
The next step is to check if the user has won, by subtracting
the selected number from the value in the count
field and comparing it to 1.
function letsgo(yourchoice) {
var date=new Date(),mychoice;
if(document.game.count.value-yourchoice<=1) {
document.game.help.value=" You
win !";
document.game.count.value=1; }
If the user has won, the help
field is updated with an appropriate message, and the single match
left is displayed in count.
Assuming the user hasn't won, the next step is to decide the computer's
next play.
else {
if(document.game.count.value%4==0) {
if(yourchoice==1) mychoice=2;
else {
if(date.getSeconds()%2==0)
mychoice=3;
else mychoice=1;
} }
To do this, see if the number of matches left was a multiple of
four before the user selected. If it was, and the user chose one
match, then the computer chooses two. Otherwise, the computer
makes a random choice between one and three matches.
else if(document.game.count.value%4==1)
mychoice=4-yourchoice;
If the remainder of dividing the number (before the user's choice)
by four is one, then the computer selects the result of subtracting
the user's choice from four.
else if(document.game.count.value%4==2)
{
if(yourchoice==2) mychoice=3;
else {
if(date.getSeconds()%2==0)
mychoice=1;
else mychoice=2;
} }
If the remainder is two, and if the user chose two, the computer
chooses three matches. Otherwise, it makes a random choice between
one and two.
else {
if(yourchoice==1) mychoice=1;
else {
if(date.getSeconds()%2==0)
mychoice=2;
else mychoice=3;
} }
If the remainder is three, and the user chose one match, the computer
follows suit and
chooses one. Otherwise, a random choice is made between two and
three.
if(document.game.count.value-yourchoice<4)
mychoice=document.game.count.value-yourchoice-1;
document.game.count.value-=yourchoice+mychoice;
document.game.me.value=" "+mychoice;
if(document.game.count.value==1) document.game.help.value=
" I
took "+mychoice+" matches and win this game !";
else document.game.help.value=
" I
took "+mychoice+" matches. It's your turn again.";
} }
Finally, if the user's play results in the number of remaining
matches being less than four, then the computer chooses the number
of remaining matches less one.
The function then updates the value of the count
field and displays the computer's choice in me.
Then a check is made to see if the computer has won. If the number
of remaining matches is one, then the computer has won, and a
message is displayed in help
to that effect. Otherwise, a message informing the user of how
many matches the computer took is displayed in help.
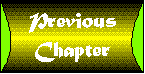 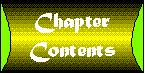 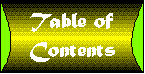 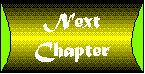
|